GCP web services used Laravel framework – Part 1
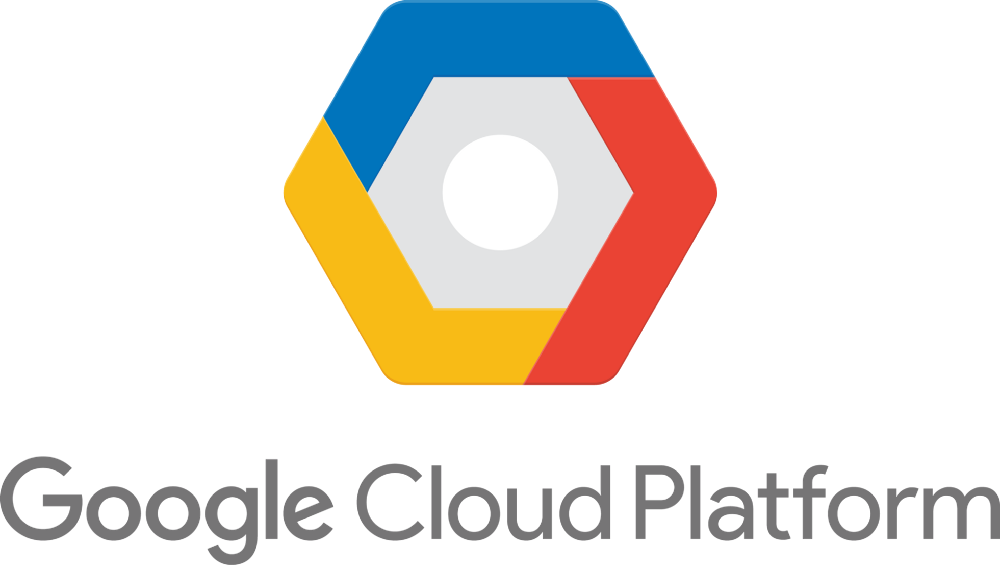
I. What is GCP?
Google Cloud Platform (GCP), offered by Google, is a suite of cloud computing services that runs on the same infrastructure that Google uses internally for its end-user products, such as Google Search, Gmail, and YouTube. Alongside a set of management tools, it provides a series of modular cloud services including computing, data storage, data analytics, and machine learning. Registration requires a credit card or bank account details.
Google Cloud Platform provides infrastructure as a service, platform as a service, and serverless computing environments.
II. Laravel Framework.
Laravel is a free, open-source PHP web framework, created by Taylor Otwell and intended for the development of web applications following the model–view–controller (MVC) architectural pattern and based on Symfony. Some of the features of Laravel are a modular packaging system with a dedicated dependency manager, different ways for accessing relational databases, utilities that aid in application deployment and maintenance, and its orientation toward syntactic sugar.
The source code of Laravel is hosted on GitHub and licensed under the terms of MIT License.
III. Run Laravel on Google App Engine (GAE) standard environment.
Before you run Laravel on GAE, you need to create a new GCP project. Log in to GCP console in this link https: https://console.cloud.google.com/
Step 1: After you, signed-in to GCP, create a project in the Google Cloud Platform Console. Click on “CREATE PROJECT” :
Step 2: There are steps to take to create a new GCP project.
Step 3: Dashboard in the newly created project
Step 4: Create a new project with the Laravel framework
Open your terminal and run command line:
– Create a new project (gcp-webservices) by composer: composer create-project --prefer-dist laravel/laravel gcp-webservices
– Go to Laravel source directory: cd gcp-webservices
– Start server localhost: php artisan serve
In your browser, visit to URL link: http://localhost:8000/
Step 5: Install and initialize the Google Cloud SDK (for MAC OS 64bit)
Open your terminal and run command line:
– Go to the downloaded directory cd google-cloud-sdk
– Install Google Cloud SDK: ./install.sh
– Login to GCP: gcloud auth login
. If gcloud
command-line not found. Please add 2 command lines below:
source "/google-cloud-sdk/completion.bash.inc"
source "/google-cloud-sdk//path.bash.inc"
– Show projects list in GCP: gcloud projects list
– Connect to gcp-webservices project: gcloud config set project gcp-webservices
– Deploy:
1. Create an app.yaml file with the following contents:
runtime: php72
env_variables:
## Put production environment variables here.
APP_KEY: YOUR_APP_KEY
APP_STORAGE: /tmp
VIEW_COMPILED_PATH: /tmp
SESSION_DRIVER: cookie
2. Go to Laravel source directory: cd gcp-webservices
3. Replace YOUR_APP_KEY in app.yaml with an application key you generate with the following command:
php artisan key:generate --show
4. Modify bootstrap/app.php
by adding the following block of code before the return statement. This will allow you to set the storage path to /tmp for caching in production.
# [START] Add the following block to `bootstrap/app.php`
/*
|--------------------------------------------------------------------------
| Set Storage Path
|--------------------------------------------------------------------------
|
| This script allows you to override the default storage location used by
| the application. You may set the APP_STORAGE environment variable
| in your .env file, if not set the default location will be used
|
*/
$app->useStoragePath(env('APP_STORAGE', base_path() . '/storage'));
# [END]
5. Go to Laravel source directory: cd gcp-webservices
6. Remove the beyondcode/laravel-dump-server
composer dependency. This is a fix for an error which happens as a result of Laravel’s caching in bootstrap/cache/services.php
.
composer remove --dev beyondcode/laravel-dump-server
7. Enable Cloud Build API here.
8. Run the following command to deploy your app: gcloud app deploy --version develop
(“develop” is the first version to deploy). Remember to delete the composer.lock file at the first deployment. Then, add composer.lock in the .gcloudignore file.
9. Visit http://YOUR_PROJECT_ID.appspot.com to see the Laravel welcome page. Replace YOUR_PROJECT_ID with the ID of your GCP project, for example, https://your-gcp-service.appspot.com/
IV. Google Vision API
Step 1: Install the Google Vision API by command line:
composer require wapnen/google-cloud-vision-php
Step 2: Create a service account key at using this instruction https://cloud.google.com/vision/docs/before-you-begin
From the Service account list, select New service account.
In the Service account name field, enter a name(in this example, it is “test“)
Then you click on “Create” to download a .json file. KEEP this file privately, if another one has it, they can use it for their private services, which you will pay $ for that.
V. Implement Google Vision API to recognize texts from images
You can refer to this main Vision document page google-vision-php
Implementation is so easy with some lines of code
With key_path you must set in .env file as an environment variable point to the path of key.json file which we generated from step IV, in this example, I will save file key.json in a folder named “google-credential” and put it in root project’s directory
and in .env include this line
Now we will create a controller to handle 2 things:
- Receive upload image from request
- Use the above code to show the text received from Vision API
I will create a controller name AnnotationController with only 1 function annotateImage() and contain the below code
I create a very simple template with only 1 input file named “Upload image” and a button submit named “Convert”
Now I will choose the above image to check if Vision can recognize the texts like “Upload image”, “Choose File”,… or not
Now check what will we get.
Amazing!! Right but now we will try with Japanese. I try with screenshot a Japanese news.
Now, what will we have?
So cool, that right?